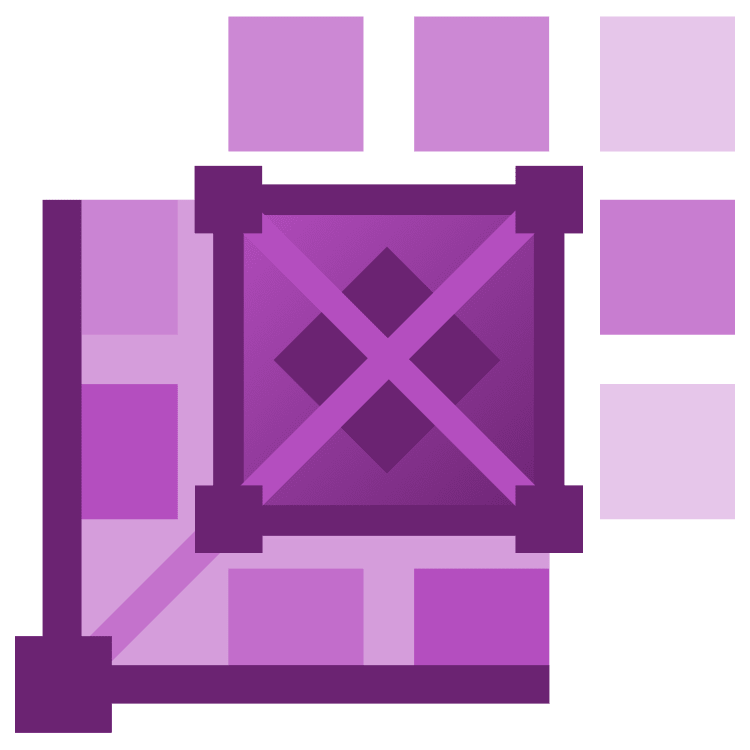
Making Responsive UI in Godot
A well-crafted responsive UI makes sure your game looks and works perfectly on any screen. Godot Engine provides a range of settings and nodes that make it easy to create responsive UIs for your game. In this tutorial, you will learn how to use these settings and nodes to create responsive UIs. By Ken Lee.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Making Responsive UI in Godot
20 mins
- Getting Started
- File Overview
- Main Scene
- Why Build Responsive UI
- Basic Knowledge of User Interface Design
- Design Resolution
- Aspect Ratio
- Orientation
- Understanding the Methods For Handling the Responsive UI
- First Approach: Keep Aspect-Ratio
- Making the Screen Align With the Player
- Using CanvasLayer to Build the Heads-Up Display
- Understanding Stretch Setting
- Exploring the Stretch Mode Setting
- Exploring the Stretch Aspect Setting
- Understanding Anchors
- Position Anchors
- Fill Anchors
- Second Approach: Filling the Window With the Content
- Improving the HUD With Anchors
- Decorating the HUD With NinePatchRect
- Building User Interface With Containers
- Creating a Health and Money Panel With GridContainer
- Adding the Health Information
- Adding the Money Information
- Switching Between Fullscreen and Window Mode
- Making Fullscreen and Window Mode Controls
- Adding the Fullscreen and Window Mode Logic
- Where to Go From Here?
Decorating the HUD With NinePatchRect
You’ve already learned how to make the existing UI responsive. Now, you’ll learn how to add a new responsive UI.
First, you’ll decorate the HUD background with a stretchable texture using the NinePatchRect node. It’s a special texture node that makes part of the texture stretch instead of the whole image.
First, create a new node of type NinePatchRect under HUD and place it above the LevelLabel. Then, rename this node to HUDBackground.
Next, drag the panel-border.png image from the File System Dock to the Texture property of HUDBackground. After that, set the anchor properties of the HUDBackground with the following values:
- Layout Mode: Anchors
- Anchor Presets: Full Rect
These settings will make the node stretch when the window is resized.
Then, set the Patch Margin properties of the HUDBackground with the following values:
- Left: 48 px
- Top: 48 px
- Right: 48 px
- Bottom: 48 px
These settings only allow the texture to stretch on the center part.
Run the game. You’ll see the background looks pretty even when the window is resized.
Building User Interface With Containers
Another tool to build a responsive UI is the Container node.
In Godot, the Container nodes manage the size and position of their child nodes. There are various containers for different layout needs.
When a Control node is a child of a Container, its layout mode is set to Container and can’t be changed.
Also, you cannot modify the Transform section’s values, like position and size, because the Container node will calculate the position and size of its children.
Creating a Health and Money Panel With GridContainer
Now, you’ll use the GridContainer node to build the Health and Money panel.
This container positions its children’s components in a grid. Use it to arrange the Health and Money information in two columns. The first column should contain icons, and the second should contain labels.
Now, start creating the container.
First, create the GridContainer node under HUD and rename the node to HealthMoneyPanel. Then, set the following properties of the node:
- Columns: 2
- Size: X = 200, Y = 80
- Layout Mode: Anchors
- Anchor Preset: Center Left
- Position: X = 40, Y = 10
You may not see any changes because there’s no child right now.
Adding the Health Information
Next, add the Health information row.
Create a TextureRect node under the HealthMoneyPanel and name it HealthIcon. Drag the life.png image to the Texture property and set the Expand Mode to Fit Width.
Then, create a Label node under the HealthMoneyPanel and name it HealthLabel. Place this node below the HealthIcon. Set the Text property to 100
and adjust the Font Size (in Control > Theme Overrides) to 30
.
Adding the Money Information
Finally, you’ll add the Money information row.
First, duplicate the HealthIcon node and rename it MoneyIcon. Then, place this node below the HealthLabel. Next, drag the coin.png image to the Texture property.
Again, duplicate the HealthLabel node. Rename it MoneyLabel. Then place this node below the MoneyIcon. Finally, set the Text property to 1000
.
You can preview the layout when you turn on the 2D view.
Run the game to check the changes. You’ll find that the Health and Money info is aligned, even when the window is resized.
This is the demo of the new layout:
Switching Between Fullscreen and Window Mode
Next, you’ll learn to switch the game to fullscreen and back to window mode. This is a common feature in desktop games because some players prefer playing in fullscreen while others like playing in window mode.
You’ll start by adding the two buttons to turn on the Fullscreen and Window mode.
Making Fullscreen and Window Mode Controls
First, create a HBoxContainer to hold the buttons and name it WindowModePanel.
Configure the properties of the WindowModePanel using the following settings:
- Size: x=100, y=48
- Layout Mode: Anchors
- Anchors Preset: Center Right
- Position: x=904, y=26
- Theme Overrides > Constants > Separation: 5
Next, add a TextureButton as a child of the WindowModePanel and rename it WindowButton.
Then, configure the WindowButton. Drag btn-window.png to the Textures (Normal) property, and set the Stretch Mode to Keep Aspect.
Duplicate the WindowButton node and rename the duplicate FullscreenButton.
Then, configure the FullscreenButton. Drag btn-fullscreen.png to the Textures (Normal) property.
Adding the Fullscreen and Window Mode Logic
Now, add the script logic to the buttons.
First, select the FullscreenButton node in the Scene tab. Set its Script property to main_scene.gd.
Then, switch to the Node tab, select the pressed() signal, and click the Connect button.
In the Connect Signal dialog, set the Receiver Method to _on_fullscreen_button_pressed
and click the Connect button.
Modify the _on_fullscreen_button_pressed
method in the main_scene.gd
script as follows:
func _on_fullscreen_button_pressed():
DisplayServer.window_set_mode(DisplayServer.WINDOW_MODE_EXCLUSIVE_FULLSCREEN)
This code uses DisplayServer.window_set_mode
to switch the window mode to fullscreen.
Next, set up the Window mode button.
First, select the node in the Scene tab for the WindowButton. Set its Script property to main_scene.gd
.
Then, switch to the Node tab. Select the pressed() signal and click the Connect button.
In the Connect Signal dialog, set the Receiver Method to _on_window_button_pressed
, and click the Connect button.
Next, change the _on_window_button_pressed
method in the main_scene.gd
script as follows:
func _on_window_button_pressed():
DisplayServer.window_set_mode(DisplayServer.WINDOW_MODE_WINDOWED)
This code invokes the DisplayServer.window_set_mode
method to switch the game back to window mode.
Now, run the game and test the functionality. You can now toggle between fullscreen and window mode using the FullscreenButton and WindowButton.
Where to Go From Here?
You now know how to create a responsive UI. Feel free to experiment with different stretch modes to suit your needs. Additionally, you can try different anchors and containers provided by the Godot engine.
For more in-depth information, check out the following articles shared by the Godot team:
If you have any questions or comments, please join the discussion below.