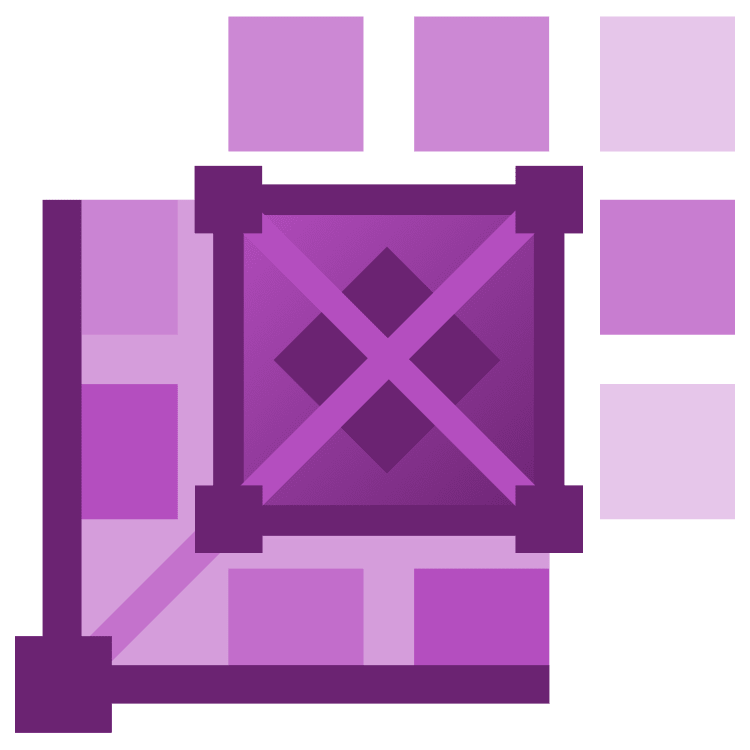
Making Responsive UI in Godot
A well-crafted responsive UI makes sure your game looks and works perfectly on any screen. Godot Engine provides a range of settings and nodes that make it easy to create responsive UIs for your game. In this tutorial, you will learn how to use these settings and nodes to create responsive UIs. By Ken Lee.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Making Responsive UI in Godot
20 mins
- Getting Started
- File Overview
- Main Scene
- Why Build Responsive UI
- Basic Knowledge of User Interface Design
- Design Resolution
- Aspect Ratio
- Orientation
- Understanding the Methods For Handling the Responsive UI
- First Approach: Keep Aspect-Ratio
- Making the Screen Align With the Player
- Using CanvasLayer to Build the Heads-Up Display
- Understanding Stretch Setting
- Exploring the Stretch Mode Setting
- Exploring the Stretch Aspect Setting
- Understanding Anchors
- Position Anchors
- Fill Anchors
- Second Approach: Filling the Window With the Content
- Improving the HUD With Anchors
- Decorating the HUD With NinePatchRect
- Building User Interface With Containers
- Creating a Health and Money Panel With GridContainer
- Adding the Health Information
- Adding the Money Information
- Switching Between Fullscreen and Window Mode
- Making Fullscreen and Window Mode Controls
- Adding the Fullscreen and Window Mode Logic
- Where to Go From Here?
With players using different screen sizes on various devices, a responsive User Interface (UI) is essential for game development. A well-crafted responsive UI ensures your game looks and works perfectly on any screen, keeping players engaged and happy. Godot Engine provides a range of settings and nodes that make creating responsive UIs for your game easy.
In this tutorial, you’ll learn how to use these settings and nodes to create responsive UIs. Here’s what you’ll learn:
- Key principles of creating a responsive UI.
- Concepts of reference resolution and aspect ratio.
You’ll also learn how to use:
- The CanvasLayer node.
- Anchor to position the nodes.
- The NinePatchRect node for backgrounds.
- GridContainer to layout children nodes.
- And how to switch between full-screen and windowed modes.
By the end of this tutorial, you’ll make the starter project’s UI responsive and add functionality to toggle between fullscreen and windowed modes.
Getting Started
This tutorial uses the most recent version of Godot 4. You can download it at Godot’s website.
Download the tutorial materials by clicking Download materials at the top or bottom of the tutorial. Then, extract the zip file to a location of your choice.
There are two projects in the materials:
- Starter: This project contains UIs without any responsive handling. You’ll convert them to responsive UIs later.
- Final: The final version of the responsive UI, which you can run and play directly.
In addition, this tutorial requires you to have basic knowledge of Godot Engine and GDScript. The following tutorials can get you up to speed:
File Overview
Import and open the starter project in Godot Project Manager. Or, double-click project.godot in the starter folder.
Take a look in the FileSystem dock and note the folders and files inside:
-
scenes: The folder that holds the scene files.
- game_map.tscn: A tile-based game map.
- player.tscn: The Player character.
- main_scene.tscn: The Main scene.
-
scripts: The scripts attached to the above scenes.
- camera_follow.gd: A Camera2D script. It makes the camera follow the Player node.
- player.gd: The script that helps you control the Player character.
- main_scene.tscn: The script that supports the main scene.
- sprites: The folder containing the graphics.
Main Scene
Time to take a look at the Main scene!
Double-click main/scene.tscn in the FileSystem dock to open the Main scene and switch to a 2D screen. You’ll see this:
Next, run the scene by pressing F5. As you can see, the game has a simple interface. You can control the player with the arrow keys to walk on the map.
The starter version does not support responsive handling, and you may notice some UI problems when you resize the window.
You’re goal is to turn this simple UI into a responsive one that looks good on all window resolutions.
Why Build Responsive UI
Responsive UI is important because players may have different devices with different screen resolutions.
Here are some common screen resolutions on different platforms:
- Desktop: 1920×1080, 1366×768, 1280×1024, 1024×768
- Mobile: 667×375, 736×414, 800×360, 844×390
- Tablet: 2732×2048, 1024×768, 601×962
Poorly managed UI responsiveness may cause issues for players and will hurt their play experience.
The following are the problems that may arise with a poorly responsive UI:
- UI elements may appear too small.
- UI elements are positioned off the screen.
- UI elements are unfilled or overlapping.
Responsive UI aims for a consistent, enjoyable experience on all screens.
Basic Knowledge of User Interface Design
Before you build a responsive UI, it’s important to learn a little about UI Design.
Next, you’ll learn about design resolution, aspect ratio, and orientation.
Design Resolution
When designing a user interface, imagine placing boxes on a canvas.
These boxes are the UI elements, like labels and buttons. The canvas is your game screen. Design resolution refers to the target dimensions of the canvas, which helps determine the width and height of UI elements to meet the design requirements.
Aspect Ratio
Aspect ratio is another important factor in UI design. It’s calculated by dividing the width of the screen by its height. By comparing the aspect ratios of your design and the screen, you’ll know if the screen is wider, narrower, or the same.
Here’s a comparison of various screen sizes and design resolutions:
Orientation
The last important factor is orientation.
There are two kinds of orientation:
- Portrait: The screen width is shorter than the screen height.
- Landscape: The screen width is longer than the screen height.
Choosing the correct orientation in the early development phase is crucial. It dramatically impacts the user interface design. For example, desktop-first games typically use landscape orientation, while it’s common for mobile-first games to use portrait orientation.
Understanding the Methods For Handling the Responsive UI
There’s two common approaches to making responsive UI.
In approach one, you keep the aspect ratio and scale all UI elements to fit the new resolution. Any remaining areas that don’t fit the screen won’t be rendered and will show as a black background.
In approach two, you adjust the aspect ratio and scale to fit the screen by using the screen’s aspect ratio. In this approach, you need to handle two scenarios:
- Define the alignment of UI elements.
- Handle the distortion of the graphical UI elements.
Next, you’ll learn how to apply these two approaches in Godot.
First Approach: Keep Aspect-Ratio
First, you’ll learn the “keep the aspect ratio” approach to handling responsive UI.
Open the Project Settings and select the Display > Window setting. Then, go to the Stretch section, set the Mode property to canvas_items and set the Aspect property to keep as its default setting.
When rerunning the game, you’ll see that the UI is responsive to the screen size change.
Here’s the result:
The UI is now no longer stuck to the top-left corner of the screen.
However, if you keep moving in the same direction, you’ll notice the player character moves out of view.
You’ll learn how to address this issue shortly.