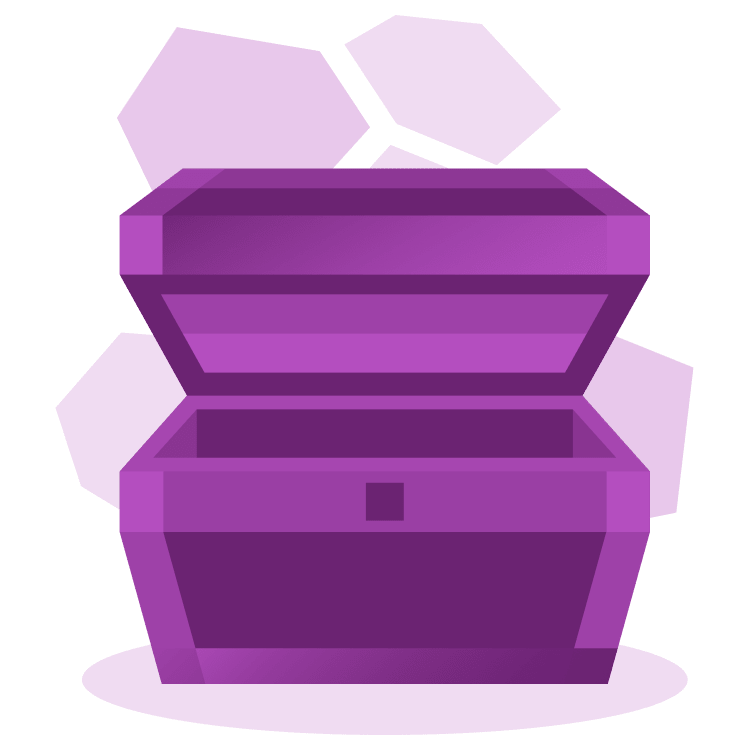
Developing for the Meta Quest 3 with Unreal Engine 5
You’ll be using Unreal Engine 5 to develop Virtual Reality on Meta Quest 3, implementing Teleportation, Grab Actions, Switches, and Deploying to Device. By Matt Larson.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Developing for the Meta Quest 3 with Unreal Engine 5
30 mins
Getting a Grip on Objects
The VRPawn provides another typical interaction — the grab action for motion controllers to hold and move an Actor after the player presses a grab button.
The grab action in the event graph finds the nearest Grab Component that might be assigned as a component within an Actor blueprint. Then, the blueprint sets the object as held by the player controller until the input is released and it becomes a free object again.
It’s time to create a grabbable Actor item.
- Open the folder Content ▸ LevelDungeon ▸ Blueprints in the Content Drawer.
- Right-click within and choose Create New Asset ▸ Blueprint Class.
- When given the option to choose what kind of Blueprint class, choose Actor.
- Name this new Blueprint class as GrabbablePotionActor.
Now, double-click to open this in the Blueprint editor and the Viewport view.
- Find the Potion_Potion model from the Content ▸ LevelDungeon ▸ ModularDungeon.
- Drag this into the Blueprint editor Components tree underneath the DefaultSceneRoot.
- Select the Potion_Potion component, and then click the Add button. Type in Grab Component and add this as a child of the Potion_Potion.
- Finish the Blueprint by clicking to Compile and then Save. Close the Blueprint editor.
You now have a Potion actor that you can interact with in VR!
Drag this into the scene at the top of the stairs and place it on the stone platform by setting the Location to X:30.0, Y:1040, Z:60.0
.
Click the Play this level in VR button to test grabbing the potion by pressing the grip buttons on the motion controller when you reach out to the potion object.
Notice, when you let go, that the potion does not fall to the ground as you might expect — it doesn’t have any gravity! Thankfully, this is easy to fix:
Return back to editing the GrabbablePotionActor, and select the Potion_Potion in the Components tree. On the details panel to the right, check to enable Simulate Physics and also check Enable Gravity.
Again, Compile and then Save and close this Blueprint editor. Now try again — this time, the potion falls to the ground on release.
Switching Gears
It’s time to dive deeper into the dungeon of VR and extend the VRPawn Blueprint to enable more complex behaviors.
A common building block for puzzles in VR is to include buttons and switches, and these devices require that the motion controller can physically interact with objects by collisions. The VRPawn lacks a physics collider on the motion controller components, so you’ll need to make changes in the blueprint.
Search the Content Drawer for the VRPawn Blueprint and double-click it to open in a Blueprint editor window.
Under the Components tab, find the HandLeft component. Choose to Add a Sphere Collision component as a child, named HandLeftCollision.
Similarly, add a Sphere Collision component, named HandRightCollision as a child under the HandRight component, further down the Components list.
Compile and Save the VRPawn Blueprint.
With collisions in-hand, you are ready to build a Blueprint for a switch.
Assembling the Lever
Begin by opening the Lever_BP Blueprint, found under the LevelDungeon ▸ Blueprints folder in the Content Drawer. This Blueprint contains a simple animated lever that will be set up to toggle with a collision:
- Start by adding a collision-sensing component to the switch. Use the Add button above Components and choose a Box Collision component to be added under the DefaultSceneRoot. Modify the Location to
X:16.0
, so that the box collision covers the lever asset as shown in the Viewport. - Switch to the Event Graph tab.
- Right-click on the Box component and choose Add Event ▸ On Component Begin Overlap to add a new element to the graph.
- Drag the arrow from the On Component Begin Overlap (Box) to add a Play Animation (lever) graph node. This automatically sets lever as the target for playing animations. You need to set the correct animation to trigger by changing New Anim to Play to use
lever_Anim
.
- Under the Variables section, add a new variable named Switch_On and make this a Boolean. Drag this into the Event Graph and choose to make it Get Switch_On value.
- Drag from it and add a NOT Boolean operator.
- Extend from the logical NOT element to add a Set Switch_On.
- Finally, connect the output arrow from Play Animation to Set Switch_On.
The resulting Event Graph should look like this:
It’s a simple blueprint, where collisions with the lever collision box activate an animation for the switch, and change the state of the switch from ‘off’ to ‘on’, or vice versa.
You’ll now make this switch affect the game itself. Save the Blueprint and close the editor.
Dropping the Gate
Next, you’ll add a dropdown gate that’s an obstacle to be controlled by the switch and prevents the player from reaching the end of the level.
Double-click the Gate_BP to open in the Blueprint editor. You’ll add logic to this Blueprint to raise the gate and then link the action of your previously built switch Blueprint to trigger this. Select the Viewport tab — you’ll see that the Gate has a Static Mesh with a child Box Collision object, as well as a NavModifier component. Together, the collider and modifier will change the available path for the player to take in the level.
The gate is an obstacle that blocks the player from teleporting into the room leading to the treasure chest. So that this gate can be a physical barrier to the player, next you must modify the Box details under Collision, to enable Simulation Generate Hit Events. Finally, set the Collision Preset as InvisibleWall.
Now, to make this gate raise and lower from Blueprint events:
- First add a Variable and name this isOpen and set it to Boolean. This will represent the state of the gate and direction of change for a Timeline to animate the gate door raising or lowering.
- Drag isOpen into the Event Graph and choose to add as Get isOpen to pull the value of the variable.
- Drag from the output arrow to add a Branch node. Connect the Event Tick to the arrow input for the node.
- The Branch node acts as an ‘if statement’, providing a True and False output. Drag from True to choose the Add Timeline… node, with True set to Play. The connect the False output to the Reverse input on the Timeline.
- The Timeline node will provide a value which you can feed as the new Z location for the gate. Double-click on the Timeline node to open in a new tab.
- Add a Track of Float Value and name this
Door_Z
. - Right-click on the Timeline graph to add a key as the starting point and give it values of
Time:0
andValue:0
. Then, add another key as an ending point withTime:3
andValue: 200
. The second point represents that the gate will move over 3 seconds to a new Z value of 200. Use the scale adjustments to get a view similar as below, or press F to quick focus:
- Return to the Event Graph tab, then drag from Update pin on the Timeline node and choose to add a Set Relative Location (SM Bars) node, automatically targeting the SM_Bars static mesh. Click on the New Location and choose to Split Struct Pin to expose individual X, Y, and Z inputs.
- Connect Door Z only to the New Location Z for movements up and down. Set the Target to the static mesh named SM_Bars to make this the target to move up and down.
Finally, you need to create an event that can be called by the Lever_BP to trigger the gate.
- Right-Click on empty space on the graph and select Add Custom Event. Name this event SetOpen and in the Details window, add an Input parameter of type Boolean called Open.
- Drag the isOpen variable onto the graph again and choose to add this time as Set isOpen.
- Connect the output of the SetOpen to the Set isOpen. Also connect the Open parameter to the isOpen variable.
- Finally, drag from the output and a new Play Sound at Location node. Select the GateRaising sound as the asset to be played.
Compare with the graph below to see if all the nodes are connected to drive the up/down movements for the gate:
Compile and Save the blueprint. Close the Blueprint Editor view to continue.