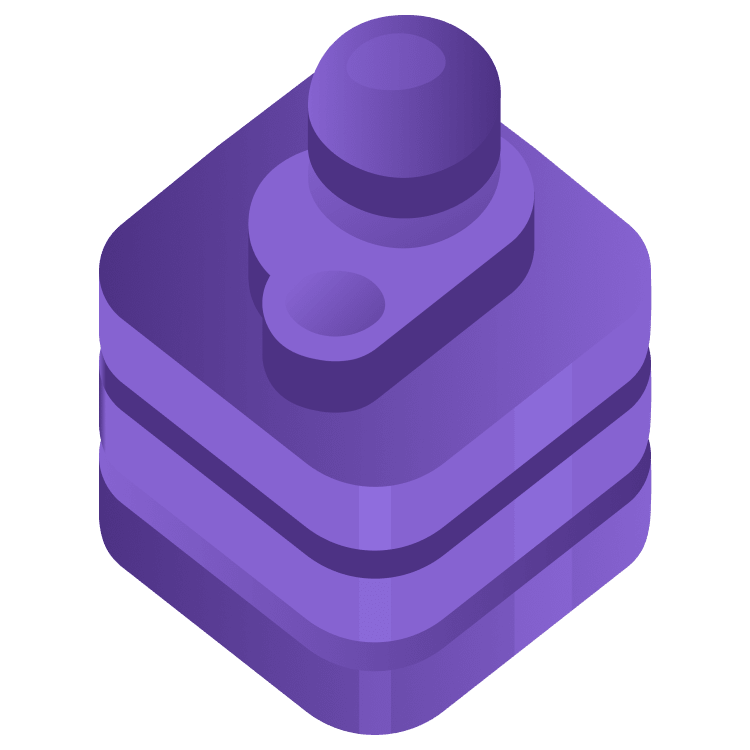
WeatherKit Tutorial: Getting Started
The tutorial covers exploring WeatherKit, displaying local weather forecasts and using Swift Charts for detailed predictions across locations. By Saleh Albuga.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
WeatherKit Tutorial: Getting Started
25 mins
- Getting Started
- Setting Up Your Project
- Registering App Identifiers
- Activating WeatherKit Capability
- Using WeatherService
- Displaying the Current Weather Forecast
- Exploring the Weather Data
- Getting a More Detailed Forecast
- 10-Day Weather Overview
- Presenting the Hourly Overview
- Adding a Daily Temperature Chart
- Adding an Hourly Temperature Chart
- Preparing the App for Distribution
- Where to Go From Here?
Preparing the App for Distribution
Many weather data providers, including WeatherKit, mandate legal attribution for their data. If you’re planning to release software using WeatherKit — be it an app or a website leveraging the WeatherKit REST API — ensure you provide the necessary attribution for the weather data. Thankfully, Apple simplifies this process for you.
Open DataAttributionView.swift and examine the code:
struct DataAttributionView: View {
var weatherAttrib: WeatherAttribution?
var body: some View {
if let attrib = weatherAttrib {
HStack {
AsyncImage(
url: attrib.squareMarkURL,
content: { image in
image.resizable()
.aspectRatio(contentMode: .fit)
.frame(maxWidth: 30, maxHeight: 30)
},
placeholder: {
ProgressView()
})
Link(destination: attrib.legalPageURL) {
Text("Weather Data Attribution")
.font(Font.footnote)
}
}
}
}
}
It’s a simple view that has one property weatherAttrib
of type WeatherAttribution
: a struct that provides the service logos and legal page URL you need.
In the view, you:
- Load, in an
AsyncImage
, thesquareMarkURL
: a square, colored Apple Weather logo. - Add a link for
legalPageURL
: a page that contains information about the weather data sources and copyright.
You’ll use this view to show the attribution. First, open CurrentWeatherView.swift. Add the following variable definition right below the currentWeather
definition:
@State var attribution: WeatherAttribution?
Then, in locationUpdated(location:error:)
, above this line:
stateText = ""
Add the following:
attribution = await weatherServiceHelper.weatherAttribution()
weatherAttribution()
is an async method that returns a WeatherAttribution
struct.
Next, in the view body
, right below the last Divider()
line, add the following:
DataAttributionView(weatherAttrib: attribution)
Build and run.
You’ll see the attribution logo and page link at the bottom. Tap the link.
You can see Apple Weather’s data sources, such as NOAA, Met Office and ECMWF.
legalPageURL
or display its contents, you can create a view showing the text in legalAttributionText
combinedMarkDarkURL
and combinedMarkLightURL
. It looks like this: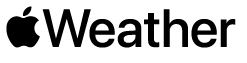
Now, you have the knowledge to copy these steps and include the attribution in DetailedWeatherView
.
Where to Go From Here?
You can download the final project by clicking the Download Materials button at the top or bottom of this tutorial.
In this tutorial, you’ve learned how to use WeatherKit to show weather forecasts in your app. You’ve acquired the skills to:
- Construct WeatherKit queries.
- Use SwiftUI Charts for forecast visualization.
- Integrate necessary data attributions for app publication.
For a deeper dive, Apple’s official resources are invaluable:
I hope you’ve enjoyed this tutorial. If you have any questions or comments, please join the forum discussion below.