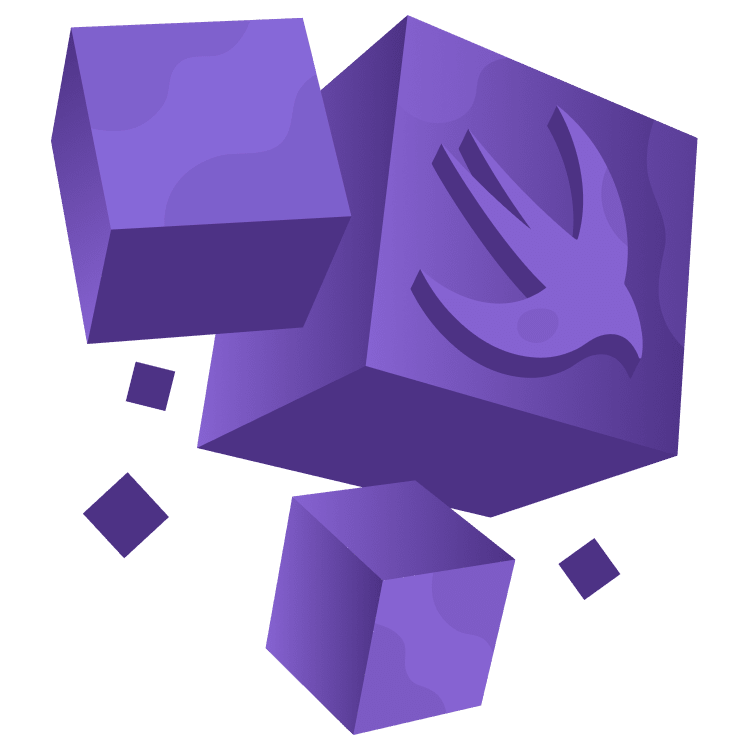
A Beginner’s Guide on Core iOS Tools
Swift and Xcode are powerful tools that enable anyone to build remarkable iOS applications. In this article, you’ll learn how to create a beginner friendly yet visually captivating iOS app, highlighting the key features and capabilities of Swift and Xcode. By Gina De La Rosa.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
A Beginner’s Guide on Core iOS Tools
10 mins
In the ever-evolving world of mobile technology, the demand for engaging and innovative iOS applications continues to grow. If you’re eager to dive into iOS development, now is the perfect time to harness the power of Swift and SwiftUI, Apple’s cutting-edge tools for building remarkable apps. In this article, we will guide you step-by-step through the creation of your first iOS application, showcasing the capabilities of Swift and SwiftUI along the way.
What Are Swift and SwiftUI?
At the heart of our iOS development journey lies Swift, Apple’s innovative and powerful programming language. Designed from the ground up to be safe, fast, and expressive, Swift has quickly become the language of choice for building apps for Apple’s platforms.
Swift’s clean syntax, robust type system, and advanced language features make it an exceptional choice for creating high-performance, reliable, and maintainable applications. By leveraging Swift’s strengths, you can write code that is not only efficient but also a joy to work with.
Complementing Swift is SwiftUI, Apple’s declarative UI framework that simplifies the process of designing and building user interfaces for iOS, iPadOS, and other Apple devices. Unlike the traditional imperative approach, where you describe how the UI should be built, SwiftUI allows you to declaratively define what the UI should look like, making it easier to create responsive and visually appealing interfaces.
The key difference between Swift and SwiftUI is that Swift is a general-purpose programming language used for the underlying logic and functionality of your iOS app, while SwiftUI is a declarative UI framework that enables you to create the visual elements and user interactions.
By combining the power of Swift and the convenience of SwiftUI, you’ll be able to build comprehensive iOS applications that not only look great but also function seamlessly. SwiftUI’s declarative approach, coupled with Swift’s performance and expressiveness, will empower you to create intuitive, responsive, and visually striking user experiences as you continue your iOS development journey.
What is Xcode?
Xcode is the essential tool that enables you to design, code, test, and submit your applications for the App Store. It’s Apple’s integrated development environment (IDE) and is crucial for the development process.
Xcode provides a comprehensive suite of features and capabilities tailored specifically for building apps for Apple platforms, including iPhone, iPad, Mac, Apple Watch, and Apple TV. From the intuitive user interface to the powerful debugging tools, Xcode streamlines the entire development workflow, allowing you to focus on bringing your creative ideas to life.
Xcode is available for macOS, and is easily installable via the App Store. You’ll need to install it to follow this article.
Building a Rotating Membership Card App
For this iOS app, we’ll create a captivating animation featuring a rotating membership card-style rectangle with curved edges and a gradient background. This project will introduce you to the core concepts of SwiftUI and demonstrate how to bring dynamic visual effects to life.
To begin, make sure you have the latest version of Xcode installed on your Mac. You can download it here, https://developer.apple.com/xcode/.
Setting Up the Project
- Open Xcode and choose “Create New Project…” from the welcome screen.
- Select “iOS” as the platform and “App” as the template, then click “Next.”
- Enter any name you like but in this case “Membership Card” also works. You don’t have to worry about adding a team account. The organization identifier can be your name for this demo.
- Select SwiftUI for the interface and Swift for the language, then click “Next.”
- Choose a location to save your project and click “Create.”
Designing the User Interface with SwiftUI
In SwiftUI, you define your user interface declaratively by describing what your app should look like, rather than imperatively describing how to build it. Let’s start by creating the rotating membership card-style rectangle.
- In the Project Navigator, open the “ContentView.swift” file.
- Replace the existing code with the following:
import SwiftUI
struct ContentView: View {
@State private var rotation: Angle = Angle(degrees: 0.0)
@State private var isAnimating = false
var body: some View {
VStack {
// Title text with formatting
Text("Membership Card")
.font(.system(size: 24, weight: .bold))
.foregroundColor(Color.white)
.frame(maxWidth: .infinity)
.padding(.top, 20)
Spacer()
// Stacked container for card
ZStack {
RoundedRectangle(cornerRadius: 16)
.fill(
// Create gradient fill with two colors
LinearGradient(
gradient: Gradient(colors: [
Color(#colorLiteral(
red: 0.5568627715,
green: 0.3529411852,
blue: 0.9686274529,
alpha: 1)),
Color(#colorLiteral(
red: 0.2392156869,
green: 0.6745098233,
blue: 0.9686274529,
alpha: 1))
]),
startPoint: .topLeading,
endPoint: .bottomTrailing
)
)
.frame(width: 300, height: 180) // Set card size
.rotation3DEffect(rotation, axis: (x: 0, y: 1, z: 0))
.onAppear {
// Animate rotation
withAnimation(.easeInOut(duration: 1.0)) {
rotation = Angle(degrees: 360.0)
isAnimating = true // Set animation played flag
}
}
}
Spacer() // Add some empty space
// Horizontal stack for slider
HStack {
Spacer() // Add space before slider
Slider(value: $rotation.degrees, in: 0...360)
.padding() // Add padding around slider
}
}
.background(Color.mint) // Set background color to mint green
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Setting Up the Basics:
The code starts by importing SwiftUI. Then, it defines a new area named ContentView
that will represent the membership card on the screen.
Keeping Track of Things (State Variables):
The code uses special variables known as @State
to remember certain things about the membership card. One variable, named rotation
, keeps track of how many degrees the card is currently rotated (initially set to 0 degrees). Another variable, named isAnimating
, remembers whether the animation has already played (initially set to false
).
Building the Membership Card View:
The main part of the code describes what the user will see on the screen. It uses a vertical stack called VStack
to arrange the elements one on top of another. At the top, there’s a text element displaying “Membership Card” in a bold white font. Below the text, there’s a spacer element that acts like a blank space to create some breathing room between elements.
The most interesting part is the membership card itself. The code creates a rectangular shape with rounded corners using RoundedRectangle
. This rectangle is filled with a beautiful gradient that smoothly transitions from a light blue to a darker blue using LinearGradient
. The code also positions the rectangle on the screen with a specific width and height called frame
and allows it to rotate in 3D space using rotation3DEffect
.
Animation Time!
When the screen appears for the first time, the code performs a neat trick. It uses a special code block triggered by the onAppear
event. This block ensures the animation only runs once upon initial view appearance. Inside this block, the code smoothly rotates the card a full 360 degrees over one second using an animation with an easeInOut
timing curve (starts slow, speeds up, then slows down to stop).
Taking Control (Slider):
While the card animates on its own, you can also play with its rotation using a slider element placed at the bottom. This slider is created using the Slider
element, and it allows you to adjust the card’s rotation to any angle between 0 and 360 degrees. The value of the slider is directly linked to the rotation
variable using two-way binding ($rotation.degrees
), so moving the slider will change the card’s rotation on the screen.
Finishing Touches:
The code defines a mint green color for the background behind the membership card, creating a pleasant contrast.
Running the App
To see your app in action click the “Play” button (the triangle icon) in the top-left corner of Xcode to build and run your app.
Xcode will compile your code, install the app on the simulator, and launch it. You should see the rotating credit card-style rectangle in action, with the user able to control the rotation using the slider.
Congratulations! You’ve just created your first iOS app using Swift and SwiftUI. This simple yet engaging animation showcases the power of Apple’s development tools and the creativity you can unleash with them.